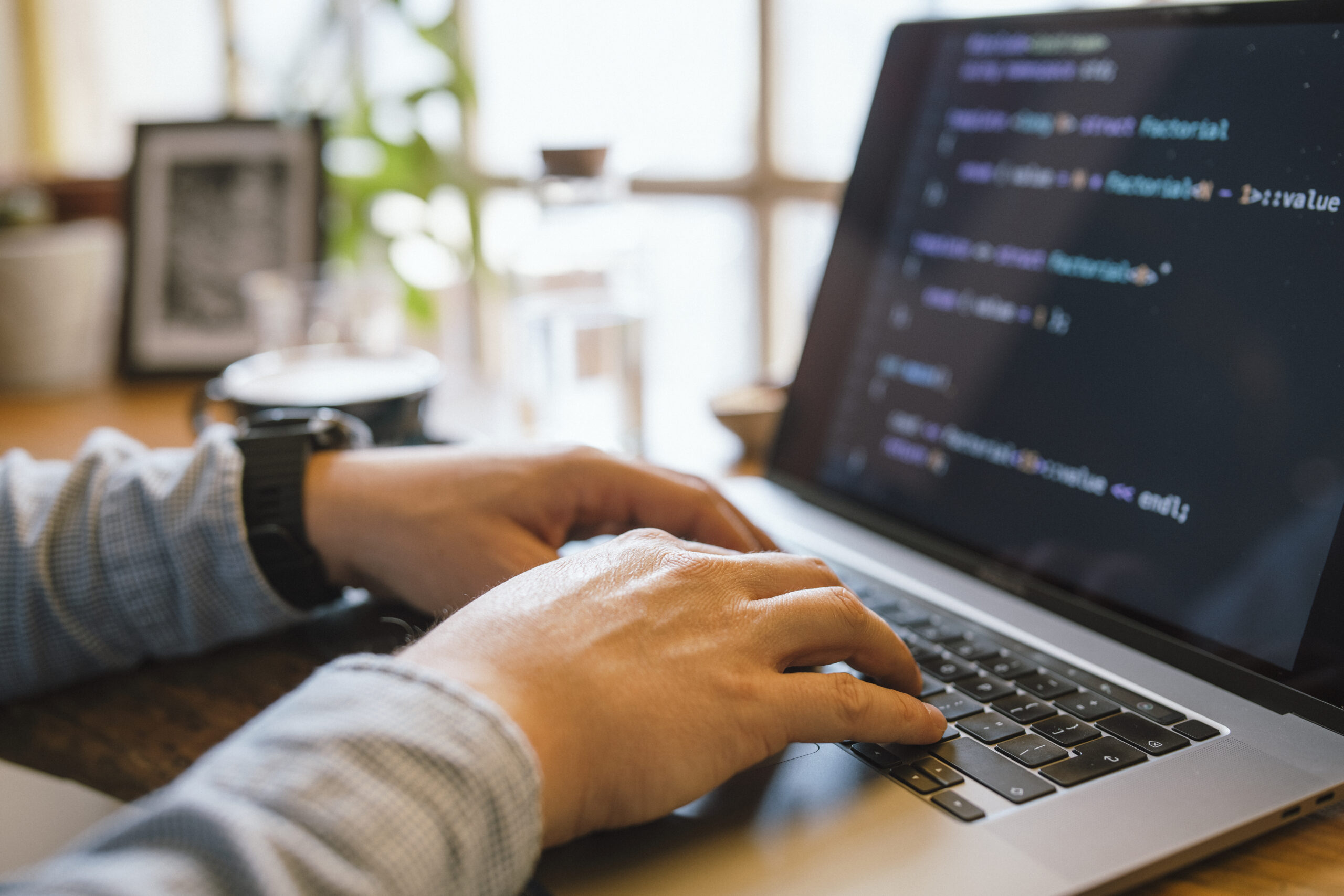
Debugging is one of the most crucial — still often ignored — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Feel methodically to resolve difficulties proficiently. No matter if you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productiveness. Listed below are a number of methods to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use every day. When producing code is a single A part of development, recognizing tips on how to communicate with it effectively all through execution is Similarly essential. Modern progress environments arrive Geared up with strong debugging capabilities — but quite a few developers only scratch the surface of what these instruments can do.
Acquire, by way of example, an Integrated Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and even modify code to the fly. When utilized the right way, they Allow you to notice specifically how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, such as Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of network requests, perspective genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, grow to be at ease with Variation control methods like Git to grasp code heritage, obtain the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates going past default options and shortcuts — it’s about building an intimate understanding of your advancement setting making sure that when difficulties occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time it is possible to shell out fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Just before jumping into your code or building guesses, developers require to produce a reliable setting or situation exactly where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, usually bringing about squandered time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you might have, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough details, try to recreate the challenge in your neighborhood atmosphere. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated assessments that replicate the edge circumstances or point out transitions involved. These exams not simply assist expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue could possibly be environment-particular — it would materialize only on certain working devices, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for tolerance, observation, and a methodical strategy. But when you finally can continuously recreate the bug, you're already halfway to fixing it. Using a reproducible situation, You need to use your debugging instruments additional proficiently, exam opportunity fixes properly, and connect extra Evidently with all your workforce or customers. It turns an abstract criticism right into a concrete obstacle — Which’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most useful clues a developer has when anything goes Mistaken. As opposed to viewing them as irritating interruptions, builders really should study to deal with error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. Quite a few developers, specially when underneath time stress, look at the primary line and right away start building assumptions. But deeper in the mistake stack or logs might lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — go through and understand them initially.
Split the error down into sections. Could it be a syntax mistake, a runtime exception, or even a logic error? Does it point to a certain file and line number? What module or purpose triggered it? These inquiries can manual your investigation and position you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake occurred. Check out similar log entries, input values, and recent improvements during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and turn into a far more economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most effective instruments in a very developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like effective begin-ups), Alert for opportunity difficulties that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the process can’t proceed.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and slow down your method. Deal with critical activities, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-imagined-out logging approach, you'll be able to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to method the method just like a detective resolving a secret. This mindset assists break down intricate challenges into workable parts and adhere to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency troubles. Similar to a detective surveys a criminal offense scene, accumulate just as much suitable facts as you could without the need of jumping to conclusions. Use logs, test circumstances, and consumer studies to piece collectively a clear picture of what’s happening.
Next, form hypotheses. Ask yourself: What could be producing this actions? Have any variations recently been made into the codebase? Has this challenge transpired just before below similar instances? The target is usually to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the trouble inside of a managed atmosphere. In case you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code queries and let the final results direct you nearer to the truth.
Spend shut awareness to modest specifics. Bugs often cover inside the least envisioned spots—like a lacking semicolon, an off-by-a single mistake, or possibly a race condition. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Lastly, hold notes on what you tried out and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for upcoming problems and enable Other people recognize your reasoning.
By thinking like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden difficulties in elaborate methods.
Publish Checks
Writing tests is one of the best solutions to help your debugging skills and All round growth efficiency. Exams not simply enable capture bugs early but will also serve as a safety net that gives you self-confidence when producing alterations to the codebase. A very well-analyzed software is much easier to debug mainly because it helps you to pinpoint accurately where and when a problem takes place.
Get started with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can promptly expose no matter if a certain piece of logic is Functioning as expected. Whenever a test fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously being preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These help make sure several areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your checks can inform you which A part of the pipeline unsuccessful and below what disorders.
Creating checks also forces you to Imagine critically about your code. To check a characteristic properly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch far more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
If you're too near the code for much too long, cognitive exhaustion sets in. You may perhaps start overlooking obvious faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less effective at problem-resolving. A brief stroll, a coffee break, or simply switching to a unique undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing valuable in the event you take some time to mirror and assess what went Completely wrong.
Start by asking by yourself some vital thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught previously with far better procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Everything you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Other folks avoid the same challenge boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, several of the very best builders aren't those who write best code, but those that repeatedly learn from their problems.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a more info bug, have a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging techniques requires time, follow, and tolerance — but the payoff is big. It would make you a far more efficient, assured, and able developer. Another time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.